Introduction to Loops and Conditionals
Pattern Making
Asking for Help in Slack
As we progress in the semester you will probably be using slack more as you ask tutors or your peers for help with programming. Here are some best practice guidelines for asking for help. There are two methods for sending code in Slack.
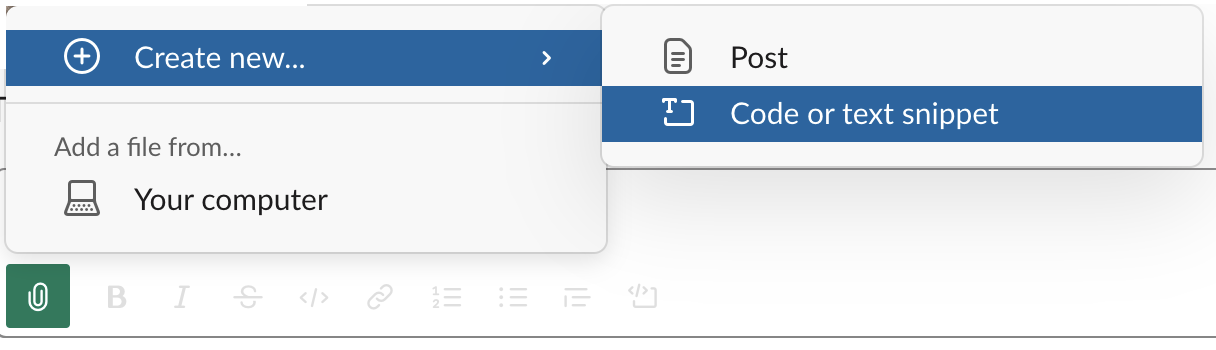
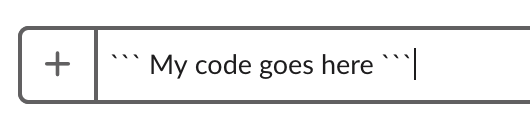

Clicking on the plus in slack and posting your code in the dialogue box.
Using three backtick keys in a row which will present your code nicely and differentiate it from what you are saying in text.
Introduction to Loops and Conditionals
Conditionals
Introduction to conditionals and expressions
-
For Loops
Introduction to for loops and key uses
-
Loops and Conditionals
Using loops and conditionals to generate patterns
Computers can make choices for us.
We call these conditionals. They are a way to choose between different instructions the program follows. In this example the conditional decides which emojis are dawn to screen based on the mouseX position.
Conditionals
Conditionals are basic test that let our software make decisions. They let actions take place only when a specific condition is met. In this simple example you can play rock paper scissors with the computer. The way this works is we tell the computer to generate a random number and draw to screen a different emoji based on that number.
In this course, we create will our own conditionals to respond to inputs with unknown visual outputs.
If Statements Elements
The if statement is the primary conditional we will use in this course. The test must be an expression that resolves to true or false.
If test evaluates to true, the statements inside the block (between
{ }
braces) will run. If the statement is not true we can use the
else
conditional to specify code to run if the test we have defined is not true.
if (test) {
// if the test is true run this code
statements
} else {
// if the first test fails run this code
statements
}
If Statement Example
Conditionals allow a program to make decisions about which lines of code should be run. They let actions take place only when a specific condition is met.
Conditionals allow a program to behave differently depending on the values of variables. For example, the program may draw a line or an ellipse depending on the value of a variable.
let x = 10;
if (x < 5) {
// The code inside this block will not run
line(10,10,50,50);
} else {
// An ellipse will be drawn
ellipse(50,50,20,20);
}
Expressions
An expression is like a phrase in English. An expression always has a value, determined by evaluating its contents.
Relational Expressions
Relational expressions return Boolean values, i.e., the result of evaluating a relational expression is either true or false.
We use these as tests for our conditionals.
Expression
Evaluation
3 > 5
false
3 < 5
true
5 < 3
false
5 > 3
true
Conditionals
Using the relational expressions as a test for a conditional statement:
if (3 < 5) {
ellipse(100, 100, 20, 20); // This code will be run
}
if (3 > 5) {
rect(20, 20, 40, 40); // This code will not be run
}
Because the test simply needs to be an expression that evaluates to true we can sometimes just use a variable that holds a true or false value. For example, the mousePressed environmental variable that is true whenever the mouse button has been pressed, so we can write code like this:
if (mouseIsPressed) {
// This code will be run when the mouse button is pressed
ellipse(100, 100, 50, 50);
}
Relational Operators
Operators used in relational expressions compare two values to calculate a relation between them, e.g., is one greater than another
Conditionals
We can use these operators to write different tests:
if (mouseX <= width/4) {
// The mouse x-coordinate is less than or equal too
// a quater of the canvas
rect(width/4, height/2, 50, 50);
}
if (mouseX >= width-width/4) {
// The mouse x-coordinate is greater than or equal too
// three quaters of the canvas
ellipse(width-width/4, height/2, 50, 50);
}
We can also test non-numeric data, for example:
function keyPressed() {
// Everytime the key a is pressed this function run
if (key == 'a') {
ellipse(100, 100, 20, 20);
}
}
Logical Expressions
Conditionals with Logical Expressions
We can combine operators to make for complex tests:
if (mouseX >= width/4 && mouseX <= (width/4)*3) {
// If the mouse x-coordinate is greater than
// or equal too a quater of the canvas
// and less than or equal to three quaters of the canvas
ellipse(width/2, height/2, 20, 20);
}
We can also combine non-numeric data, for example:
function keyPressed() {
if (key == 'a' || key == 'A') {
// If either a or A is pressed
// Draw an ellipse to screen
ellipse(width/2, height/2, 20, 20);
}
}
Loops / Iterations
For Loops
A for loop runs through a block of code a set amount of times. The block of code that is looped is grouped together in what we call a block, this block is delimited by
{
to start and
}
to end.
For loops are made up of three statements on the same line:
for (init; test; update) {
// Initalise a variable
// Test if condition is true
// If true run statement statements
// Update variable
}
Using For Loops for Iteration
Iteration can be used to greatly reduced the amount of code required to accomplish repetitive tasks. The two code snippets bellow have the exact same output.
line(50, 50, 50, 500);
line(100, 50, 100, 500);
line(150, 50, 150, 500);
line(200, 50, 200, 500);
line(250, 50, 250, 500);
line(300, 50, 300, 500);
line(350, 50, 350, 500);
line(400, 50, 400, 500);
line(450, 50, 450, 500);
line(500, 50, 500, 500);
for (let i = 50; i <= 500; i += 50) {
// Variable 'i' is declared
// Loop checks that 'i' is less than or equal to 500
// If this is true, a line is drawn
// Once complete 'i' is increased by 50
// We can use this varaible 'i' to help us draw to screen efficiently
line(i, 50, i, 500);
}
Nested For Loops
Using nested for loops allow us to create coordinates for shapes with very little repetition of code. We can create a grid of shapes with minimal lines of code, this makes it fast to make major changes to these patterns.
In this course, we regularly pair for loops with the
map
function so we can keep ours for loops as simple as possible.
// Our ellipses will start at coordinates 100, 100 and iterate
// Over every loop to create a grid of ellipses
for(let x = 100; x < width; x += 100) {
// the code inside here loops as many times at the for loop above it
for(let y = 100; y < height; y += 100) {
// The code in here loops a multiple of both loops together
// If outside loop runs 5 times and the inner loop runs 6 times
// The code here will run 5 x 6 = 30 times
ellipse(x, y, 50);
}
}
The Map Funciton
The
map
function converts a range of numbers into a
different range of numbers. An example of where this would be
helpful is when we are working with dynamic variables such as
mouseX. This is an environment variable that gives us numbers as
large as the width of the canvas as it is simply returning the
current mouse position on the canvas. We may want something to
follow our mouse and change color as well but since our all of
our color functions only accept values between 0 and 255 we must
use the map function to change the range of number returned from
mouseX
.
noStroke();
// Here we take mouseX as an input, measure it from
// 0 to width then turn those numbers into 100 to width - 100
// You'll notice that the ellipse will follow the mouse but
// Stop short of the end of the canvas
let xPos = map(mouseX, 0, width, width/4, (width/4)*3);
// Here we are doing the same as above however
// We are turning mouseX coordinates into color friendly numbers
let mappedColor = map(mouseX, 0, width, 0, 255);
fill(mappedColor);
ellipse(xPos, height/2, 50);
Map Function and Loops
Pairing loops with the map function solves three key problems we face when we are drafting our generative designs.
- We often don't know the exact dimensions of the canvas.
- Allowing for flexibility for changes in the design while keeping elements even and centered.
- Reference positions in an array (this is a future problem).
// this loop will always run 5 times
for (let i = 0; i < 5; i++) {
// this remaps the variable of the loop into a proportionate x coordinate
let xPos = map(i, 0, 4, 100, width - 100);
ellipse(xPos, height / 4, 50);
}
// this loop will run an unknown amount of times
for (let x = 100; x < width; x += 100) {
ellipse(x, (height / 4) * 3, 50);
}
Using For Loops with Color
We can extend this relationship between the for loop and the mapping function to help us calculate color relative to coordinate. In this example below, there is a line being drawn at every y coordinate until the bottom. 0 to the of the canvas height is an unknown distance so we can remap this number range to something we can use for our color functions (0 to 255).
function setup() {
createCanvas(windowWidth, windowHeight);
}
function draw() {
// Create a for loop that loops for every coordinate on the y axis
for(let i = 0; i < height; i++) {
// Create a variable which remaps value from the for loop
let colorChanging = map(i, 0, height, 255, 0);
stroke(colorChanging, 100, 200);
line(0, i, width, i);
}
}
The Modulus Operator %
The modulus operator is our way to divide a number and see if it
has a remainder. This is helpful when we are trying to apply a
style or draw something if our variable tested is divisible by a
certain number. In this example we are testing if 'i' from our
for loop is divisible by two, effectively asking the program to
do something for us every time the for loop is on an even
number. For example
10 % 2 == 0
resolves to true,
as we are asking when the number 10 is divided by two does it
leave a remainder of 0, in other words, is 10 divisible by two.
Note that in this example that our for loop starts at 0 so the first line will be red.
for(let i = 0; i < 10; i++) {
let xPos = map(i, 0, 9, 100, width-100);
// Divides 'i' by 2 and determines if there are any remainders
// If there are no remainders it is divisible by 2
// As a result, this operator resolves at true every time 'i' is even
if(i % 2 == 0) {
// Even numbers would be drawn in red
stroke(255,0,0);
} else {
stroke(20);
}
line(xPos, 0, xPos, height);
}